The Copy & Paste Hell-Loop
Copy & paste has always been seen as bad practice but let's be honest, we all do it.
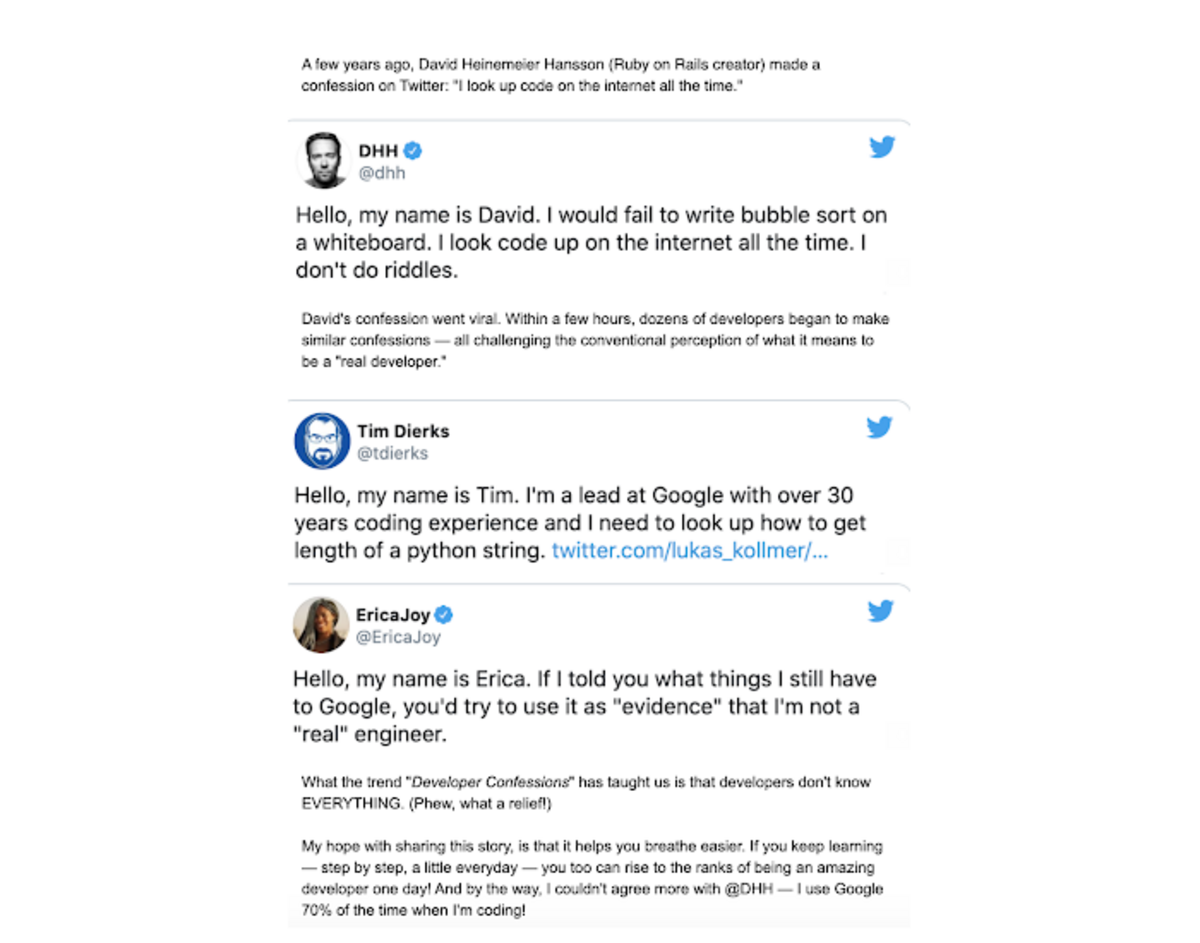
Especially in the dev community, copy & paste is very common - as is looking things up, because we are not computers and we don’t know everything. But, we’ve learned that there are limitations to copy & paste when it comes to code (ok well, to everything, not only code, but you’ll see what I mean). Lines of code are easy to copy & paste, but you should always pay attention to certain things to avoid those lines ruining your project.
Be lazy, not efficient!
Sometimes we are too overwhelmed and stressed from deadlines, so we feel smart by (re-)using code that's already been written, or we just feel too lazy to (re-)type all those lines of code...so why not just copy & paste, right?
Wrong! I can assure you that you will want to bite your past self when it comes down to evaluating or refactoring the copy & paste code. Why, you ask? Let’s look at an example.
Let's say we have some php code to append a string to another string and uppercase the result:
```
$aString = "a string";
$anotherString = " and another string";
$resultString = $aString . $anotherString;
echo $resultString; // outputs "a string and another string"
```
This is an easy one to copy & paste; it’s too many lines that we would need to rewrite over and over again throughout our project. So let’s copy & paste those lines everywhere we need to concat and uppercase two strings!
OH NO WAIT!
We forgot to uppercase the result. No problem at all, with Find & Replace (Ok, that could end up you in another hell, but let's save it for another article). Now the refactored code looks like this:
```
$aString = "a string";
$anotherString = " and another string";
$resultString = strtoupper($aString . $anotherString);
echo $resultString; // outputs "A STRING AND ANOTHER STRING"
```
Easy enough.
Be efficient?
But, wait! Now the project description has changed.
We need to check the code and see if the second string is not the same as the first one before concatenating both, because if they are the same,we don't need to concatenate them.
OR, maybe the second string needs further manipulation? Or, perhaps the whole code needs a complete logic makeover?
I hope you see where this ends, or rather, you realise the never ending loop you’ve just pasted into your project and its aftermath, requiring you to change all these tiny pieces of code.
But, no need to give up (yet)! There might be a solution called "functions". You create a function like this :
```
function concatString($stringA, $stringB) {
return strtoupper($stringA . $stringB);
}
$aString = "a string";
$anotherString = " and another string";
$resultString = concatString($aString, $anotherString);
echo $resultString; // outputs "A STRING AND ANOTHER STRING"
```
This is a great solution: You only need to change the logic of the code in the `concatString` function and may call `concatString` everywhere you need to concat two strings and uppercase the result in your project.
It is a good thing to have "functions'' and now you can use it in all your new projects. And how will you use it? Most likely by copy & pasting the function from one project to another.
The functional efficiency dilemma
As time goes by, you’ve created dozens of projects and almost forgot what the function `concatString` looks like. You simply copy and paste it from some other project into this one. What could go wrong? `concatString` always did the job for you.
Only this time, the resulting string shouldn't be in uppercase in one specific output. So you remove `strtoupper` from the function `concatString`. Suddenly you notice that every other call of `concatString` will not uppercase the resulting string . You probably end up tweaking these lines of code. Or maybe you just go looking for new lines of code to copy & paste into the existing lines of code. Welcome back to your copy & paste hell: between all those lines of code you have written around and between `concatString` might be lines of code that are not needed or do the wrong thing. You will probably end up debugging every single line of code you have written and copy & pasted so far. Every. Single. Line.
Think twice
I understand that this small self-written code of mine is rather stupid as an example. So let's go back to a real life situation.
You need some lines of code to do some stuff. So you search online or maybe in an older project and find the perfect gem of code. It looks good and seems to do the trick. You copy the gem and paste it in your new code. Job done!
Now rethink about the scenarios described earlier with the perfect gem instead of the simple code example...? Hopefully now you get me and the issues related to Copy & Paste code. Whether it is your own or someone else's code. The gem was in fact not as perfect and only shiny. The gem doesn't work or maybe does more, or other things, than it should. Or the gem may be deprecated in the future. So, was this gem really worth it when, in reality, you have to tweak and manipulate it and lose a ton of time in the process? Didn’t I tell you in the beginning that you’ll want to bite your past self for doing this? Maybe it is easier to just write that code yourself and get it right the first time around, without having to worry about any issues or unwanted results?
So, to recap, you should always understand the context where the code is taken out from and understand the code itself. Since every written code is meant for some specific task and may not always fit your project's needs.
Conclusion
Lesson (hopefully) learned: Trust, but verify.
The copy & paste code may give you the wanted results, at first, but you should always verify and understand it before pasting. You could save yourself a lot of headache this way. Or, this code could lead you to another, more useful one, and make you realise what code you actually want to write, or even teach you something for future codes.
Now then, should you copy & paste code?
The answer is: you can (as long as you check it), because reality is that everyone does it and it’s normal. Google is your friend. And there are many brilliant minds out there, posting codes that can teach you something, inspire you or take you on a ride in your copy & paste hell. The choice is yours.